6966
How to extract and apply LAS classification RGB values to a point cloud using the programming interface (API)
The following example code show how to access the classification values in a LAS file and create a new project file that will display the point cloud by the classification RGB values. This example uses the default Computed Classification Values described in the ENVI Lidar help. The values may vary depending on the LAS file version:
Computed
Classification Value
|
Description
|
0
|
Not processed
|
1
|
Unclassified
|
2
|
Terrain
|
3
|
Near terrain
|
5
|
Trees
|
6
|
Buildings
|
14
|
Power line
|
15
|
Power pole
|
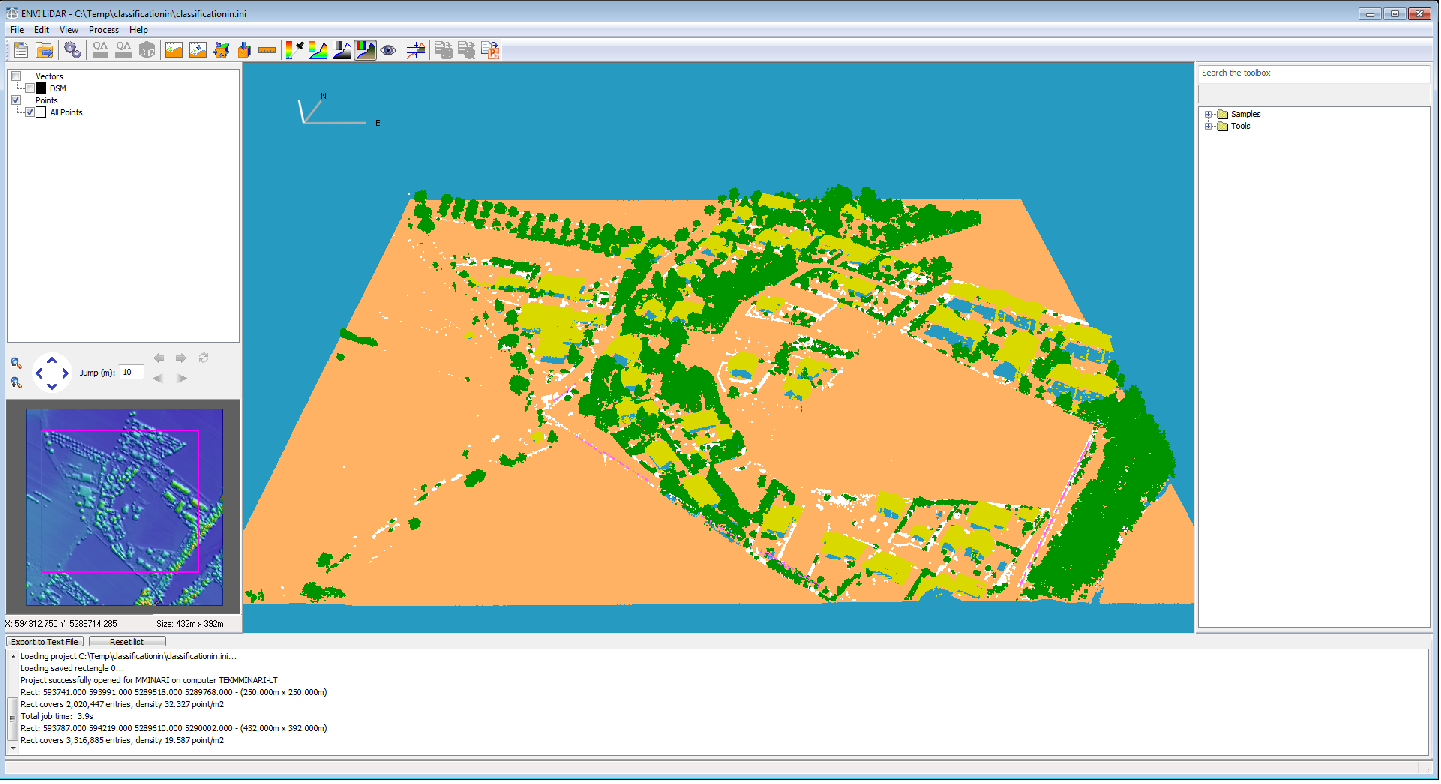
;----------------------------------------------------
PRO setRGBbyClassificationStoredInLAS
COMPILE_OPT idl2
e = e3de(/headless)
;lasFile has classification values already written to it
lasFileName = 'classificationIn.las'
;create a project from the las file
oLidar = e.openlidar(lasFileName)
startIndex = 0
blockSize = 500000
allClass = MAKE_ARRAY(blockSize, /BYTE)
allread = 0
;Loop through all points, setting the RGB value based on the stored
;classification values of the points
WHILE (allread eq 0) DO BEGIN
pointsInRange = oLidar.GetPointsInRange(startIndex, blockSize, $
ALL_ATTRIBS=ALL_ATTRIBS, $
COUNT=nPointsRead)
;inClass holds the Classifications for this iteration of the While loop
inClass = *ALL_ATTRIBS.CLASSIFICATIONS
IF (nPointsRead LT blocksize) THEN allread = 1
;allClass holds all of the Classifications in the file
allClass = [allClass,inClass]
;uniqAllClass is an array containing one entry for each unique Classification
uniqAllClass = allClass[UNIQ(allClass, SORT(allClass))]
rgb = MAKE_ARRAY(3,nPointsRead, /BYTE)
FOREACH i, uniqAllClass DO BEGIN
CASE i OF
;Add all possible product types - powerpole, nearterrain… setting the rgb values to the corresponding classification color.
;Unprocessed
0: BEGIN
index = WHERE(inClass EQ 0)
red=190
green=190
blue=190
rgb[0,index] = red
rgb[1,index] = green
rgb[2,index] = blue
END
;Unclassified
1: BEGIN
index = WHERE(inClass EQ 1)
red=255
green=255
blue=255
rgb[0,index] = red
rgb[1,index] = green
rgb[2,index] = blue
END
;Terrain
2: BEGIN
index = WHERE(inClass EQ 2)
red=255
green=177
blue=100
rgb[0,index] = red
rgb[1,index] = green
rgb[2,index] = blue
END
;Near Terrain
3: BEGIN
index = WHERE(inClass EQ 3)
red=128
green=255
blue=0
rgb[0,index] = red
rgb[1,index] = green
rgb[2,index] = blue
END
;Trees
5: BEGIN
index = WHERE(inClass EQ 5)
red=0
green=147
blue=0
rgb[0,index] = red
rgb[1,index] = green
rgb[2,index] = blue
END
;Buildings
6: BEGIN
index = WHERE(inClass EQ 6)
red=217
green=217
blue=0
rgb[0,index] = red
rgb[1,index] = green
rgb[2,index] = blue
END
;Power lines
14: BEGIN
index = WHERE(inClass EQ 14)
red=255
green=100
blue=255
rgb[0,index] = red
rgb[1,index] = green
rgb[2,index] = blue
END
;Power poles
15: BEGIN
index = WHERE(inClass EQ 15)
red=160
green=80
blue=0
rgb[0,index] = red
rgb[1,index] = green
rgb[2,index] = blue
END
ELSE: PRINT, 'Bad value'
ENDCASE
ENDFOREACH
startIndex += nPointsRead
ENDWHILE
e.CLOSE
END
;--------------------------------
MM June 12, 2015