Note: For updates to third-party libraries, see the IDL Release Notes inside the IDL installation.
New Features
Dark Mode for Windows Widgets
On Windows, IDL widgets now support a dark mode theme. You can change the theme using a new IDL preference, IDL_THEME, which can be set to 0 for light mode (the default) or 1 for dark mode. The theme preference can be set using the preferences dialog in the IDL Workbench, or using the PREF_SET routine. A new read-only system variable, !THEME, lets you determine the current value of the preference.
Some general notes:
-
On Windows, all IDL widget applications, including ENVI, will honor the theme setting.
-
If you change the theme, you must restart your IDL session for the changes to take effect.
-
The IDL widget theme is independent from the Windows system theme setting.
-
The IDL Workbench has its own theme setting that is unrelated to this setting.
-
The graphics within direct and object graphics windows are unaffected.
-
Linux and Mac platforms will quietly ignore the theme preference and will always use light mode.
Spherical Harmonic Transforms
IDL now has built-in functions to perform the forward and inverse spherical harmonic transform.
The SPHERE_HARM_FORWARD function uses the Gauss-Legendre algorithm to compute the forward spherical harmonic transform of a two-dimensional input array on a spherical coordinate grid. The SPHERE_HARM_INVERSE function computes the inverse spherical harmonic transform, and returns the result on a spherical coordinate grid. Both the forward and inverse transform are written in C code and are multi-threaded for maximum performance.
The spherical harmonic transform is a useful tool for analyzing wave patterns on a global dataset, and is extensively used in meteorology and oceanography, and in the study of geomagnetic and solar magnetic fields. The forward and inverse transforms are also useful for applying a spectral filter. In this case, the forward transform is performed, then a filtering function is applied to the spectral coefficients, and then the inverse transform is performed.
In addition to the transform functions, IDL now has two new routines: SPHERE_HARM_FILTER and SPHERE_HARM_KERNEL. These routines are useful for spectrally filtering a spherical dataset to keep wavenumbers longer than a certain threshold.
For example, here we load some air temperature data from 1 Dec 1884 and then compute the T63 truncation:
restore, filepath('reanl20v3_1dec1884_512x256dbl.sav', $
subdir=['examples', 'data'])
T63 = sphere_harm_filter(A, 63)
im = image(A, rgb_table = 75, layout=[1, 2, 1], $
dim=[550, 580], min = -30, max = 30)
im = image(T63, rgb_table = 75, /current, $
layout=[1, 2, 2])
These routines are based on the original transform and filtering routines written by Mark Miesch, Marc DeRosa, and Gilbert P. Compo, and are used with permission. For a more detailed example as well as data credits and reference, see SPHERE_HARM_FORWARD.
GAUSS_QUAD_LEGENDRE function
The new GAUSS_QUAD_LEGENDRE function computes the x values (the abscissas) and weighting coefficients for Gaussian quadrature integration, where the x values are the roots of the Legendre polynomial of order N. GAUSS_QUAD_LEGENDRE is based on the routine gauleg described in section 4.5 of Numerical Recipes in C: The Art of Scientific Computing (Second Edition), published by Cambridge University Press, and is used by permission.
Read and Convert Float16/Binary16 Half-Precision Floats
Half-precision floating-point numbers are 16 bits in length, compared to 32-bits for single-precision floats and 64-bit double precision. The standard format for these numbers is known as float16 (or binary16), and is based on the IEEE 754 standard. Although IDL does not have native support for 16-bit floats, IDL can now read and convert float16 numbers. The READ_BINARY function has a new FLOAT16 keyword which will automatically read in float16 numbers from a file and convert them to 32-bit floats. In addition, you can use the new FLOAT16_DECODE and FLOAT16_ENCODE functions to convert 16-bit unsigned integers (in float16 format) to and from 32-bit floats.
For example:
a = uint([0, 1, 0x8001, 0x3ff, 0x3c00, 0xbc00, 0x7bff, 0x7c00, 0xfc00, 0x7fff])
b = float16_decode(a)
help, b
print, b
IDL prints:
B FLOAT = Array[10]
0.00000 5.96046e-08 -5.96046e-08 6.09756e-05 1.00000 -1.00000 65504.0 Inf -Inf NaN
Now convert back:
c = float16_encode(b)
help, c
print, c, format='(10z)'
IDL prints:
C UINT = Array[10]
0 1 8001 3ff 3c00 bc00 7bff 7c00 fc00 7fff
See FLOAT16_DECODE, FLOAT16_ENCODE, and READ_BINARY for details.
Command Line Progress Bar
The new CLI_Progress class implements a command line progress bar in the IDL Workbench console or when running IDL in a terminal. For example:
length = 1000
cli_progress.initialize, title = 'Download', maximum = length, /remaining
for i=0,length do begin & $
cli_progress.update, i & $
wait,0.01 & $
endfor
Here, the progress bar will slowly advance from 0% to 100%, similar to this:
Download 46% [##################----------------------] 6m:27s
The CLI_Progress class has options for controlling the bar width, the characters, the time remaining, and the title and text message. You can also construct a "ping pong" marquee progress bar where the progress indicator bounces back and forth along the bar. For example:
CLI_Progress.Initialize, ping_pong = !true, complete_char = "<IDL>"
for i = 0,114 do begin & $
CLI_Progress.Update & $
wait, 0.02 & $
endfor
The word <IDL> bounces back and forth:
[-----------------------------------<IDL>-----]
For more information see CLI_Progress or visit the CLI_Progress examples page.
IDL_HASHVAR Function
IDL has a new IDL_HASHVAR function, which takes any IDL variable and returns a scalar 32-bit hash code representing the value. The IDL_HASHVAR function is designed for creating simple hash code values, and is useful for converting arbitrary values into 32-bit (four byte) hash codes with a uniform distribution. It can also be used to determine if two strings are identical, as a slight difference in the strings (say a single character) will product completely different hashes. For more details see IDL_HASHVAR.
New SVG Icons
IDL now ships with over 2000 new icons from the Font Awesome project. The icons can be found in the following directories in the IDL distribution:
<IDL_DIR>/resource/bitmaps/svg/brands
<IDL_DIR>/resource/bitmaps/svg/regular
<IDL_DIR>/resource/bitmaps/svg/solid
You can use the new SVG_ICON_BROWSER to display all of the icons, and you can use the new RENDER_ICON and RENDER_SVG functions to access them. All of these icons are distributed under the Creative Commons Attribution 4.0 International License and can be freely used in your own applications.
RENDER_SVG Function
IDL has a new RENDER_SVG function that can render SVG files into a bitmap image with an optional output size, background color, and foreground color. For example:
file = filepath('folder-open.svg', subdir=['resource', 'bitmaps', 'svg', 'regular'])
img = RENDER_SVG(file, width=128, height=128, background=0xCCCCCC, foreground=0xFF0000)
help, img
i = image(img)
IDL prints:
IMG BYTE = Array[128, 128, 3]
RENDER_ICON Function
IDL has a new RENDER_ICON function that creates icons from SVG files in the IDL distribution. For example: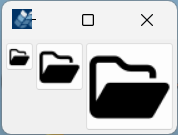
w = widget_base(/row)
icon = RENDER_ICON("folder-open")
w1 = widget_base(w)
wb = widget_button(w1, value=icon, /bitmap)
icon = RENDER_ICON("folder-open", width=32, height=32)
w1 = widget_base(w)
wb = widget_button(w1, value=icon, /bitmap)
icon = RENDER_ICON("folder-open", width=64, height=64)
w1 = widget_base(w)
wb = widget_button(w1, value=icon, /bitmap)
widget_control, w, /realize
SVG_ICON_BROWSER Routine
IDL has a new SVG_ICON_BROWSER procedure that displays a thumbnail of all 2000+ icons that ship with IDL. The browser has a filter, and also displays sample code for individual icons plus a quick look of how that icon will appear in various widget types. See SVG_ICON_BROWSER for details.
Updates
IDL Extension for VS Code
IDL now has a new, modern developer environment, available for free within Visual Studio Code. The IDL for VSCode extension can be easily downloaded and installed from the VS Code extensions page. The extension has the following features:
-
A full-featured IDL code editor, with variable type detection, auto complete, chromacoding, problem reporting, and code formatting.
-
An integrated debugger with breakpoints, or run command-line IDL within the VS Code terminal.
-
Support for an IDL Notebook-style interface, with embedded graphics and save to PDF.
-
Customizable themes and colors.
-
Hover help contains complete IDL documentation including code examples.
-
Create routine-definition documentation for routines within SAVE files or DLMs.
-
Native multi-language support.
For details visit the IDL for VSCode page.
HDF5 now handles empty arrays
The H5D_READ and H5_GETDATA routines can now handle H5T_VLEN datatypes that have zero-length arrays. In these cases, the result is a pointer to a !NULL variable.
HttpRequest Progress Bar
The HttpRequest class (introduced in IDL 9.0) now has an optional progress bar on the ::Get, ::Post, and ::Put methods. This progress bar uses the new CLI_Progress bar described above. See HttpRequest::Get, HttpRequest::Post, and HttpRequest::Put for details.
IDL_String::Replace new Count argument
The IDL_String Replace method now has a new optional Count argument. The argument lets you specify how many matches to replace. The default is to replace all matches. For example:
IDL> str = '.......'
IDL> print, str.replace('.', '*', 3)
***....
You can also specify a negative count to start from the end of the string:
IDL> str = '.......'
IDL> print, str.replace('.', '*', -3)
....***
For more details see IDL_String::Replace.
JSON support for NaN and Infinity values
IDL's JSON_PARSE function now supports both trailing commas on arrays as well as the special NaN, Inf, and Infinity (unquoted) values. These are all non-standard additions to the JSON specification, but provide better interoperability with Python and other tools that produce JSON.
In addition, JSON_SERIALIZE now has a new NANINF_LITERAL keyword. The default behavior is to output NaN and Infinity values as quoted strings, which obeys the JSON specification but does not allow them to be converted back to floating-point values. Setting the NANINF_LITERAL keyword will output these values without the quotes. This allows JSON_PARSE and other tools such as Python to understand these values and return floating-point NaN and Infinity values.
LEGEND Fill Color
You can now specify the fill color for the LEGEND graphics function:
p = plot(/test)
l = legend(target=p, fill_color='yellow')
See LEGEND for details.
MapContinents Shapefiles
The MapContinents graphics function has been updated with more up-to-date shapefiles for the continents, countries , lakes, islands, and rivers, including new high-resolution versions for continents, lakes, and rivers. For example:
m0 = Map('Geographic', limit=[41, -93, 50, -83], margin=[0.1, 0.1, 0.1, 0.05])
m0.mapgrid.label_position = 0
m0.mapgrid.linestyle = "none"
m0.mapgrid.grid_longitude = 2
m0.mapgrid.grid_latitude = 2
m1 = MapContinents(/hires, fill_color="light green")
m2 = MapContinents(/lakes, /hires, fill_color='light blue', linestyle=0)
m3 = MapContinents(/islands, fill_color='green')
m4 = MapContinents(/rivers, /hires, color='blue', thick = 2)
Multiline Tooltips
IDL widgets now support tooltips that span multiple lines. To create multi-line tooltips, simply embed newline \n characters when setting the TOOLTIP keyword. For details see the TOOLTIP keyword on WIDGET_BUTTON, WIDGET_DRAW, or WIDGET_TREE.
PRINT/PRINTF new NEWLINE keyword
The PRINT and PRINTF procedures have a new NEWLINE keyword. The default is 1, which outputs a newline (\n) character at the end of the output, as before. Setting NEWLINE=0 will suppress the newline, and allows you to call PRINT multiple times and have all of the output appear on the same line.
Shapefile Enhancements
The Shapefile library used by the IDLffShape class was updated to the latest version. In addition, IDLffShape now handles the Date and Logical attribute types. You can also retrieve the Shapefile types for all attributes with a new property. For details see IDLffShape.
TS_SMOOTH new NAN keyword
The TS_SMOOTH function has a new NAN keyword. Set this keyword to cause the routine to check for occurrences of the IEEE floating-point values NaN or Infinity in the input data. Elements with the value NaN or Infinity are treated as missing data. As such, the routine will replace these missing elements with the smoothed value of the valid points within the smoothing window (using a simple boxcar smooth).
WIDGET_BROWSER Support
WIDGET_BROWSER has been updated to include support for macOS.
WIDGET_LABEL and WIDGET_TEXT Text and Background Colors
WIDGET_LABEL and WIDGET_TEXT now support the FOREGROUND_COLOR and BACKGROUND_COLOR keywords, which let you set the text color and the background text box color. You can also use WIDGET_CONTROL to change these properties dynamically. For example, save and run the following code:
pro widtext_ex_change, ev
common _widtext, w1, w2
widget_control, w1, foreground_color=bytscl(randomu(s,3)), background_color=bytscl(randomu(s,3))
widget_control, w2, foreground_color=bytscl(randomu(s,3)), background_color=bytscl(randomu(s,3))
end
pro widtext_ex
common _widtext, w1, w2
w = widget_base(xsize = 200, /column)
w0 = widget_base(w, /row)
f = 'Segoe*24'
w1 = widget_label(w0, value='Label', foreground_color=[0,200,0], background_color=[255,255,0], font=f, scr_xsize=100)
w2 = widget_text(w0, value='My Text', /editable, foreground_color=[200,0,0], background_color=[0,255,255], font=f)
w0 = widget_base(w, /row)
wbutton = widget_button(w0, value='Change', event_pro = 'widtext_ex_change', font=f)
widget_control, w, /realize
xmanager,'widtext_ex', w, /no_block
end
WIDGET_PROPERTYSHEET Top Header Keyword
WIDGET_PROPERTYSHEET has a new TOP_HEADER keyword that when set to zero hides the top (column) header.
WIDGET_TEXT Alignment Keywords
WIDGET_TEXT has six new alignment keywords:
-
The ALIGN_BOTTOM, ALIGN_CENTER, ALIGN_LEFT, ALIGN_RIGHT, and ALIGN_TOP keywords control the placement of the widget within its parent base. These keywords are allowed on all platforms. The default is ALIGN_CENTER.
-
The new ALIGNMENT keyword allows you to set the text alignment. This keyword is available for Microsoft Windows and is quietly ignored on other platforms.
WIDGET_TEXT FRAME=0
On Microsoft Windows, for WIDGET_TEXT you can now set FRAME=0 to remove the border around the text widget. On other platforms, text widgets always have a frame and setting FRAME=0 will have no effect.
WRITE_PNG Compression Keyword
The WRITE_PNG function has a new COMPRESSION keyword which lets you control the amount of compression for the PNG output. The value must be in the range 0 to 9. A value of 0 is no compression. Higher values will give smaller files but will be slower to write and read. The default is 6, which gives a good balance between compression and speed.
Dataminer Installation
The Dataminer module is now installed with IDL by default. It still requires a license for use. Dataminer supports reading from and connecting to Microsoft Excel, PostgreSQL, and a number of other database management systems.
See Also
See What's New (Previous IDL Releases) for an archive of What's New information.