You can shrink or expand one-, two-, or three-dimensional image arrays using the CONGRID and REBIN functions. CONGRID resizes an array by any arbitrary amount, and REBIN resizes by an integer multiple of the original dimensions.
For more information on how these functions work, see How Image Arrays are Resized.
Resizing to a Specific Size
The following example uses the CONGRID function. This example data is available in the examples/data directory of your IDL installation. The code shown below creates the graphics shown here.
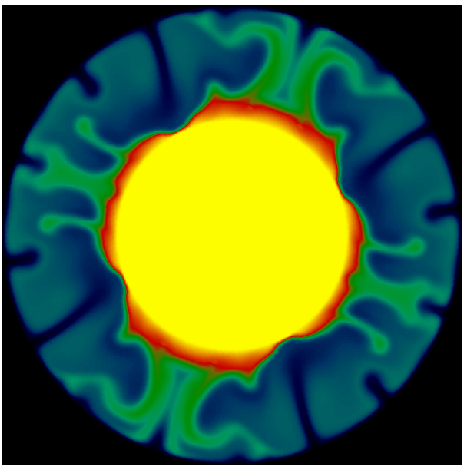
Copy the entire code block and paste it into the IDL command line to run it.
file = FILEPATH('convec.dat', SUBDIRECTORY=['examples', 'data'])
data = READ_BINARY(file, DATA_DIMS=[248, 248])
im = IMAGE(data, RGB_TABLE=28)
magnifiedIm = CONGRID(data, 600, 600, /INTERP)
im2 = IMAGE(magnifiedIm, RGB_TABLE=28)
Resizing to a Multiple of the Image Array Size
You can achieve similar results using REBIN, but you need to specify a multiple of the original array size.
The following example uses the same data as the previous example, using REBIN to resize the image display:
file = FILEPATH('convec.dat', SUBDIRECTORY=['examples', 'data'])
convec_image = READ_BINARY(file, DATA_DIMS=[248, 248])
im = IMAGE(convec_image, RGB_TABLE=28)
rebinIm = REBIN(convec_image, 744, 744)
im2 = IMAGE(rebinIm, RGB_TABLE=28)
How Image Arrays are Resized
When expanding an image array, new values are interpolated from the source image to produce additional pixels in the output image array:
- CONGRID defaults to nearest-neighbor sampling with one- or two-dimensional arrays and automatically uses bilinear interpolation with three-dimensional arrays.
- REBIN defaults to bilinear interpolation.
When shrinking an image array, IDL resamples pixels to produce a lower number of pixels in the output image array:
- CONGRID uses nearest-neighbor interpolation to resample the array.
- REBIN averages neighboring pixel values in the source image that contribute to a single pixel value in the output image.
Resources